import { Rating } from "@/components/ui/rating"
const Demo = () => {
return <Rating defaultValue={3} size="sm" />
}
Setup
If you don't already have the snippet, run the following command to add the
rating
snippet
npx @chakra-ui/cli snippet add rating
The snippet includes a closed component composition for the RatingGroup
component.
Usage
import { Rating } from "@/components/ui/rating"
<Rating />
Examples
Sizes
Use the size
prop to change the size of the rating component.
import { Stack } from "@chakra-ui/react"
import { Rating } from "@/components/ui/rating"
const Demo = () => {
return (
<Stack>
<Rating defaultValue={3} size="xs" />
<Rating defaultValue={3} size="sm" />
<Rating defaultValue={3} size="md" />
<Rating defaultValue={3} size="lg" />
</Stack>
)
}
Controlled
Use the value
and onValueChange
prop to control the rating value.
"use client"
import { Rating } from "@/components/ui/rating"
import { useState } from "react"
const Demo = () => {
const [value, setValue] = useState(3)
return <Rating value={value} onValueChange={(e) => setValue(e.value)} />
}
ReadOnly
Use the readOnly
prop to make the rating component read-only.
import { Rating } from "@/components/ui/rating"
const Demo = () => {
return <Rating readOnly defaultValue={3} size="sm" />
}
Hook Form
Here's an example of how to use rating with react-hook-form
.
"use client"
import { Button, Stack } from "@chakra-ui/react"
import { zodResolver } from "@hookform/resolvers/zod"
import { Field } from "@/components/ui/field"
import { Rating } from "@/components/ui/rating"
import { Controller, useForm } from "react-hook-form"
import { z } from "zod"
const formSchema = z.object({
rating: z.number({ required_error: "Rating is required" }).min(1).max(5),
})
type FormValues = z.infer<typeof formSchema>
const Demo = () => {
const {
handleSubmit,
formState: { errors },
control,
} = useForm<FormValues>({
resolver: zodResolver(formSchema),
})
const onSubmit = handleSubmit((data) => console.log(data))
return (
<form onSubmit={onSubmit}>
<Stack gap="4" align="flex-start">
<Field
label="Rating"
invalid={!!errors.rating}
errorText={errors.rating?.message}
>
<Controller
control={control}
name="rating"
render={({ field }) => (
<Rating
name={field.name}
value={field.value}
onValueChange={({ value }) => field.onChange(value)}
/>
)}
/>
</Field>
<Button size="sm" type="submit">
Submit
</Button>
</Stack>
</form>
)
}
Custom Icon
Use the icon
prop to pass a custom icon to the rating component. This will
override the default star icon.
import { Rating } from "@/components/ui/rating"
import { IoHeart } from "react-icons/io5"
const Demo = () => {
return (
<Rating
colorPalette="red"
icon={<IoHeart />}
allowHalf
count={5}
defaultValue={3.5}
/>
)
}
Half Star
Use the allowHalf
prop to allow half-star ratings.
import { Rating } from "@/components/ui/rating"
const Demo = () => {
return <Rating allowHalf defaultValue={3.5} />
}
Emoji
Compose the rating component with emojis.
import { RatingGroup } from "@chakra-ui/react"
const emojiMap: Record<string, string> = {
1: "😡",
2: "😠",
3: "😐",
4: "😊",
5: "😍",
}
const Demo = () => {
return (
<RatingGroup.Root defaultValue={3}>
<RatingGroup.Control>
{Array.from({ length: 5 }).map((_, index) => (
<RatingGroup.Item
key={index}
index={index + 1}
minW="9"
filter={{ base: "grayscale(1)", _checked: "revert" }}
transition="scale 0.1s"
_hover={{ scale: "1.1" }}
>
{emojiMap[index + 1]}
</RatingGroup.Item>
))}
</RatingGroup.Control>
</RatingGroup.Root>
)
}
Testimonial
Use the rating component to show testimonials.
Sage is a great software engineer. He is very professional and knowledgeable.
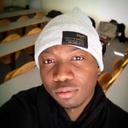
Matthew Jones
CTO, Company
import { HStack, Stack, Text } from "@chakra-ui/react"
import { Avatar } from "@/components/ui/avatar"
import { Rating } from "@/components/ui/rating"
const Demo = () => {
return (
<Stack maxW="320px" gap="4">
<Rating colorPalette="orange" readOnly size="xs" defaultValue={5} />
<Text>
Sage is a great software engineer. He is very professional and
knowledgeable.
</Text>
<HStack gap="4">
<Avatar
name="Matthew Jones"
src="https://randomuser.me/api/portraits/men/70.jpg"
/>
<Stack textStyle="sm" gap="0">
<Text fontWeight="medium">Matthew Jones</Text>
<Text color="fg.muted">CTO, Company</Text>
</Stack>
</HStack>
</Stack>
)
}
Props
Root
Prop | Default | Type |
---|---|---|
count | '5' | number The total number of ratings. |
colorPalette | 'gray' | 'gray' | 'red' | 'orange' | 'yellow' | 'green' | 'teal' | 'blue' | 'cyan' | 'purple' | 'pink' | 'accent' The color palette of the component |
size | 'md' | 'xs' | 'sm' | 'md' | 'lg' The size of the component |
allowHalf | boolean Whether to allow half stars. | |
asChild | boolean Use the provided child element as the default rendered element, combining their props and behavior. For more details, read our Composition guide. | |
autoFocus | boolean Whether to autofocus the rating. | |
defaultValue | number The initial value of the rating group when it is first rendered. Use when you do not need to control the state of the rating group. | |
disabled | boolean Whether the rating is disabled. | |
form | string The associate form of the underlying input element. | |
id | string The unique identifier of the machine. | |
ids | Partial<{
root: string
label: string
hiddenInput: string
control: string
item(id: string): string
}> The ids of the elements in the rating. Useful for composition. | |
name | string The name attribute of the rating element (used in forms). | |
onHoverChange | (details: HoverChangeDetails) => void Function to be called when the rating value is hovered. | |
onValueChange | (details: ValueChangeDetails) => void Function to be called when the rating value changes. | |
readOnly | boolean Whether the rating is readonly. | |
required | boolean Whether the rating is required. | |
translations | IntlTranslations Specifies the localized strings that identifies the accessibility elements and their states | |
value | number The current rating value. | |
as | React.ElementType The underlying element to render. | |
unstyled | boolean Whether to remove the component's style. |
Item
Prop | Default | Type |
---|---|---|
index * | number | |
asChild | boolean Use the provided child element as the default rendered element, combining their props and behavior. For more details, read our Composition guide. |